Calculating discounts in Visual Basic is a common task in many programming scenarios, such as e-commerce applications or financial analysis tools.
Understanding how to calculate discounts accurately is crucial for ensuring correct calculations and avoiding potential errors.
This article will provide a comprehensive guide to calculating discounts in Visual Basic, covering the relevant formulas, code examples, and best practices.
How to Calculate Discount in Visual Basic
Discount calculations are an essential aspect of many programming applications, such as e-commerce and financial analysis. Understanding the key aspects of discount calculation in Visual Basic is crucial for accurate and efficient programming.
- Formulas
- Code examples
- Best practices
- Common errors
- Debugging techniques
- Performance considerations
These aspects cover various dimensions of discount calculation in Visual Basic, from theoretical formulas to practical implementation and troubleshooting. By understanding these aspects, programmers can develop robust and reliable applications that handle discounts accurately and efficiently.
Formulas
Formulas are the cornerstone of discount calculations in Visual Basic. They provide the mathematical framework for determining the discounted price based on the original price and the discount rate. Here are several key aspects of formulas in relation to discount calculation:
- Discount Percentage Formula
This formula calculates the discount amount as a percentage of the original price. It is expressed as Discount = Original Price * Discount Rate.
- Discount Amount Formula
This formula directly calculates the discount amount in monetary terms. It is expressed as Discount = Original Price – Discounted Price.
- Discounted Price Formula
This formula calculates the discounted price by subtracting the discount amount from the original price. It is expressed as Discounted Price = Original Price – Discount.
- Compound Discount Formula
This formula is used when multiple discounts are applied sequentially. It calculates the overall discounted price by applying the discounts in a cumulative manner.
These formulas provide a comprehensive set of tools for performing accurate discount calculations in Visual Basic. Understanding and applying these formulas is essential for developing robust and reliable applications that handle discounts effectively.
Code examples
Code examples are indispensable to understanding how to calculate discounts in Visual Basic. They provide concrete illustrations of the concepts and formulas involved, making it easier for programmers to grasp the practical implementation of discount calculations. Without code examples, the theoretical aspects of discount calculation would remain abstract and difficult to apply in real-world scenarios.
Real-life examples of code examples include:
- A simple console application that calculates the discounted price of an item based on a given discount percentage.
- A Windows Forms application that allows users to enter the original price and discount rate, and displays the discounted price.
- A class library that provides a set of methods for performing various discount calculations, such as calculating the discount amount, the discounted price, and the compound discount.
Understanding the connection between code examples and how to calculate discounts in Visual Basic is crucial for developing robust and reliable applications that handle discounts accurately and efficiently. By studying and experimenting with code examples, programmers can gain a deeper understanding of the underlying concepts and best practices, and apply them effectively in their own projects.
Best practices
In the context of calculating discounts in Visual Basic, best practices refer to a set of guidelines and techniques that help ensure accuracy, efficiency, and maintainability in your code. By adhering to best practices, you can develop robust and reliable applications that handle discounts effectively.
One of the key best practices is to use clear and descriptive variable names. This makes it easier to understand the purpose of each variable and reduces the risk of errors. For example, instead of using a variable named “d” to store the discount amount, you could use a more descriptive name like “discountAmount”.
Another best practice is to use appropriate data types for your variables. For example, if you are storing the discount rate as a percentage, you should use a data type that supports decimal values, such as the Decimal data type. This ensures that you can accurately represent the discount rate and avoid potential rounding errors.
By following best practices, you can improve the quality and reliability of your code, making it easier to maintain and debug in the future. Additionally, adhering to best practices can help you avoid common pitfalls and errors, such as using incorrect formulas or data types.
Common errors
Understanding common errors associated with discount calculations in Visual Basic is critical for avoiding potential pitfalls and ensuring accurate results. These errors can arise from various factors, including incorrect formulas, data type mismatches, or logical mistakes.
- Incorrect formula usage
Errors can occur when using incorrect formulas or applying them inappropriately. For instance, using the discount percentage formula to calculate the discount amount instead of the discount amount formula can lead to incorrect results.
- Data type mismatches
Mismatches between data types can also cause errors. For example, attempting to apply a discount percentage to a non-numeric value or using an incorrect data type for the discount amount can lead to exceptions or incorrect calculations.
- Logical errors
Logical errors occur when the code contains incorrect logic or assumptions. For instance, failing to handle special cases, such as negative discount rates or zero prices, can result in unexpected behavior.
- Round-off errors
When working with floating-point numbers, round-off errors can occur due to the limited precision of these data types. This can lead to slight inaccuracies in discount calculations, especially when dealing with small values or multiple discounts.
By understanding and addressing these common errors, developers can improve the accuracy and reliability of their discount calculation code in Visual Basic. It is important to thoroughly test and validate the code to ensure that it handles various scenarios correctly and produces the expected results.
Debugging techniques
Debugging techniques play a crucial role in ensuring the accuracy and reliability of discount calculations in Visual Basic. Without proper debugging, errors and inconsistencies in the code can lead to incorrect or unexpected results, compromising the integrity of the application. Debugging techniques provide a systematic approach to identifying and resolving these issues, enabling developers to pinpoint the root cause of errors and implement appropriate fixes.
One of the key benefits of debugging techniques is their ability to identify logical errors in the code. For instance, if a discount calculation is not producing the expected results, debugging techniques can help determine whether the error lies in the formula itself or in the way the formula is being applied. By stepping through the code line by line and examining the values of variables, developers can isolate the source of the error and make necessary adjustments.
In addition, debugging techniques can help identify and resolve data-related issues. For example, if a discount calculation is failing due to invalid input data, debugging techniques can help identify the source of the invalid data and implement appropriate error handling mechanisms. This ensures that the application can gracefully handle unexpected data and provide meaningful error messages to users.
Overall, debugging techniques are an essential component of discount calculation in Visual Basic, enabling developers to identify and resolve errors, improve the accuracy of calculations, and ensure the overall reliability of the application. By leveraging debugging techniques effectively, developers can build robust and dependable applications that handle discounts efficiently and accurately.
Performance considerations
Performance considerations play a critical role in discount calculations within Visual Basic. Discount calculations involve mathematical operations, data manipulation, and logical evaluations, which can impact the overall performance of the application, especially when dealing with large datasets or complex calculations. Optimizing the performance of discount calculations is essential for ensuring a responsive and efficient user experience.
One of the key performance considerations is the choice of data structures and algorithms. Selecting appropriate data structures, such as arrays or hash tables, can significantly affect the efficiency of data retrieval and manipulation during discount calculations. Similarly, choosing efficient algorithms for performing mathematical operations, such as optimized multiplication or division routines, can improve the overall performance.
Real-life examples of performance considerations in discount calculations include optimizing the calculation of compound discounts. Compound discounts involve applying multiple discounts sequentially, which can lead to performance bottlenecks if not handled efficiently. Implementing efficient algorithms for compound discount calculations, such as dynamic programming or memoization techniques, can greatly improve the performance and scalability of the application.
Understanding the connection between performance considerations and discount calculations in Visual Basic empowers developers to create high-performing applications that handle discounts accurately and efficiently. By optimizing data structures, algorithms, and leveraging appropriate techniques, developers can ensure that their applications can handle large datasets, complex calculations, and real-time requirements without compromising performance or user experience.
Frequently Asked Questions about Discount Calculations in Visual Basic
This section addresses commonly asked questions and provides clear and concise answers to help you better understand how to calculate discounts in Visual Basic.
Question 1: What is the most accurate formula for calculating discounts in Visual Basic?
The most accurate formula depends on the specific calculation you need to perform. The discount percentage formula, discount amount formula, and discounted price formula are commonly used for different scenarios. Choose the formula that aligns with your calculation requirements.
Question 2: How can I handle errors that may arise during discount calculations?
Utilize robust error handling mechanisms to trap and manage errors effectively. Implement try-catch blocks to capture exceptions, provide informative error messages to users, and ensure the stability of your application.
Question 3: What are the performance implications of discount calculations, and how can I optimize them?
Performance considerations are crucial for large datasets and complex calculations. Optimize your code by selecting efficient data structures, utilizing optimized algorithms, and employing techniques like memoization to enhance performance and scalability.
Question 4: How do I calculate compound discounts in Visual Basic accurately?
Implement efficient algorithms for compound discount calculations, such as dynamic programming or memoization techniques. These techniques help avoid redundant calculations, improve performance, and ensure accurate results, especially for complex discount scenarios.
Question 5: What are some common pitfalls to avoid when calculating discounts in Visual Basic?
Be cautious of incorrect formula usage, data type mismatches, logical errors, and round-off errors. Use appropriate data types, validate inputs, and thoroughly test your code to mitigate potential issues and ensure reliable discount calculations.
Question 6: How can I improve the readability and maintainability of my discount calculation code?
Utilize clear and descriptive variable names, follow coding conventions, and add well-structured comments to enhance code readability. This makes your code easier to understand, maintain, and debug, promoting long-term code quality.
These FAQs provide valuable insights into the nuances of discount calculations in Visual Basic. Understanding these aspects will empower you to write robust, efficient, and maintainable code that accurately handles discounts in your applications.
Moving forward, let’s delve into advanced techniques for handling complex discount scenarios, such as tiered discounts and dynamic pricing strategies.
Tips to Enhance Discount Calculations in Visual Basic
This section provides practical tips to elevate your discount calculation skills in Visual Basic, ensuring accuracy, efficiency, and maintainability.
Tip 1: Leverage the Correct Formula
Choose the appropriate formula based on your calculation needs. The discount percentage formula, discount amount formula, and discounted price formula serve different purposes.
Tip 2: Handle Errors Gracefully
Implement robust error handling to manage exceptions and provide informative error messages. This ensures application stability and user-friendliness.
Tip 3: Optimize for Performance
Consider data structures and algorithms for efficiency. Utilize optimized multiplication and division routines to enhance performance, especially with large datasets.
Tip 4: Master Compound Discounts
Employ efficient algorithms like dynamic programming or memoization for compound discount calculations. This prevents redundant calculations and ensures accuracy.
Tip 5: Avoid Common Pitfalls
Be aware of potential errors such as incorrect formula usage, data type mismatches, logical errors, and round-off errors. Validate inputs and thoroughly test your code.
Tip 6: Enhance Code Readability
Use descriptive variable names, follow coding conventions, and add well-structured comments. This promotes code clarity and maintainability.
Tip 7: Utilize Debugging Techniques
Employ debugging techniques to identify and resolve errors efficiently. Step through your code and examine variable values to pinpoint the root cause of any issues.
Tip 8: Consider Special Cases
Handle special cases like negative discount rates or zero prices gracefully. Implement logical checks to ensure your code behaves as expected in all scenarios.
By following these tips, you can significantly improve the accuracy, efficiency, and maintainability of your discount calculation code in Visual Basic.
In the next section, we will delve into advanced techniques for handling complex discount scenarios, such as tiered discounts and dynamic pricing strategies, to further enhance your discount calculation capabilities.
Conclusion
This comprehensive guide has delved into the intricacies of discount calculation in Visual Basic, providing a solid foundation for developers to accurately and efficiently handle discounts in their applications. By understanding the key formulas, best practices, and debugging techniques, developers can write robust and reliable code that meets the demands of real-world scenarios.
Throughout this exploration, several key points have emerged:
- Choosing the right formula is crucial for accurate discount calculations, whether it’s the discount percentage formula, the discount amount formula, or the discounted price formula.
- Handling errors gracefully through robust error handling mechanisms ensures the stability and user-friendliness of the application.
- Optimizing for performance by considering efficient data structures, algorithms, and techniques like memoization can significantly improve the efficiency of discount calculations, especially with large datasets.
By embracing these insights and continuously honing their skills, developers can elevate their discount calculation capabilities, creating applications that are not only accurate but also efficient and maintainable. The significance of mastering discount calculations in Visual Basic extends beyond the immediate task at hand; it empowers developers to build feature-rich and user-centric applications that cater to the diverse needs of modern software systems.
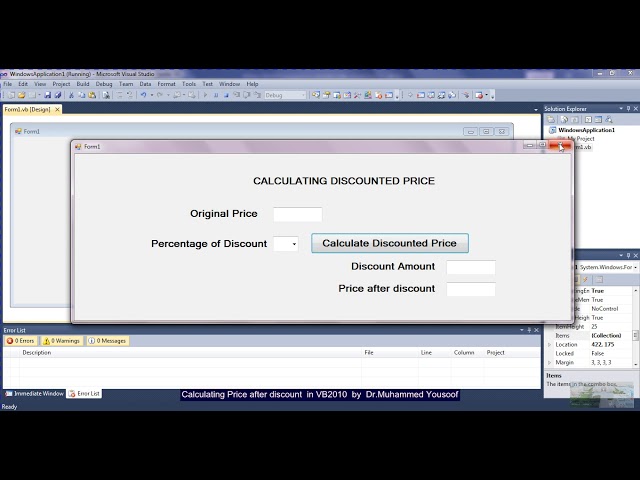